C# 문법
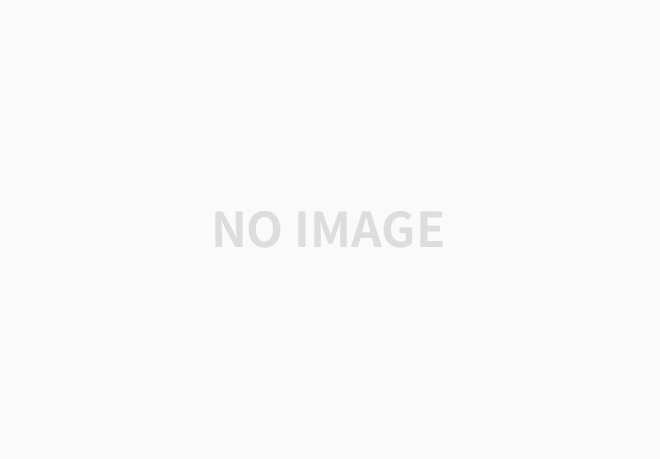
global using System;
using System.Globalization;
using static System.Console;
namespace Hello
{
class MainApp
{
//enum DialogResult { YES, NO, CANCEL, CONFIRM, OK };
enum DialogResult { YES = 10, NO, CANCEL, CONFORM = 50, OK };
static void Main(string[] args)
{
//1번 테스트
/*
if (args.Length == 0)
{
Console.WriteLine("사용법 : Hello.exe <이름>");
return;
}
WriteLine("Hello, {0}!", args[0]);
*/
//2번 테스트
/*
sbyte a = -10;
byte b = 40;
Console.WriteLine($"a={a}, b={b}");
short c = -30000;
ushort d = 60000;
Console.WriteLine($"c={c}, d={d}");
int e = -1000_0000; //0이 7개
uint f = 3_0000_0000; // 0이 8개
Console.WriteLine($"e={e}, f={f}");
long g = -5000_0000_0000; //0이 11개
ulong h = 200_0000_0000_0000_0000; //0이 18개
Console.WriteLine($"g={g}, h={h}");
*/
//3번 테스트
/*
byte a = 240; //10진수 리터럴
Console.WriteLine($"a={a}");
byte b = 0b1111_0000; //2진수 리터럴
Console.WriteLine($"b={b}");
byte c = 0XF0; //16진수 리터럴
Console.WriteLine($"c={c}");
uint d = 0x1234_abcd; //16진수 리터럴
Console.WriteLine($"d={d}");
*/
/*
byte a = 255;
sbyte b = (sbyte)a;
Console.WriteLine(a);
Console.WriteLine(b);
*/
/*
uint a = uint.MaxValue;
Console.WriteLine(a);
a = a + 1;
Console.WriteLine(a);
*/
/*
float a = 3.1415_9265_3589_7932_3846f;
Console.WriteLine(a);
double b = 3.1415_9265_3589_7932_3846;
Console.WriteLine(b);
*/
/*
float a = 3.1415_9265_3589_7932_3846_2643_3832_79f;
double b = 3.1415_9265_3589_7932_3846_2643_3832_79;
decimal c = 3.1415_9265_3589_7932_3846_2643_3832_79m;
Console.WriteLine(a);
Console.WriteLine(b);
Console.WriteLine(c);
*/
/*
char a = '안';
char b = '녕';
char c = '하';
char d = '세';
char e = '요';
Console.WriteLine(a);
Console.WriteLine(b);
Console.WriteLine(c);
Console.WriteLine(d);
Console.WriteLine(e);
Console.WriteLine();
*/
/*
string a = "안녕하세요\n할룽";
string b = "김원호입니다";
Console.WriteLine(a);
Console.WriteLine(b);
string multiline = """
별 하나에 추억과
별 하나에 사랑과
별 하나에 쓸쓸함과
별 하나에 동경과
별 하나에 시와
별 하나에 어머니, 어머니
""";
Console.WriteLine(multiline);
string aaa = """
fsdf
sdf
sdf
""";
Console.WriteLine(aaa);
*/
/*
bool a = true;
bool b = false;
Console.WriteLine(a);
Console.WriteLine(b);
*/
/*
object a = 123;
object b = 3.141592653589793238462643383279m;
object c = true;
object d = "안녕하세요";
Console.WriteLine(a);
Console.WriteLine(b);
Console.WriteLine(c);
Console.WriteLine(d);
*/
/*
int a = 123;
object b = (object)a; //a에 담긴 값을 박싱해서 힙에 저장
int c = (int)b; //b에 담긴 값을 언박싱해서 스택에 저장
Console.WriteLine(a);
Console.WriteLine(b);
Console.WriteLine(c);
double x = 3.1414213;
object y = x; //x에 담긴 값을 박싱해서 힙에 저장
double z = (double)y; //y에 담김 값을 언박싱해서 스택에 저장
Console.WriteLine(x);
Console.WriteLine(y);
Console.WriteLine(z);
*/
/*
sbyte a = 127;
Console.WriteLine(a);
int b = (int)a;
Console.WriteLine(b);
int x = 128; //sbyte의 최대값 127보다 1큰수
Console.WriteLine(x);
sbyte y = (sbyte)x;
Console.WriteLine(y);
*/
/*
float a = 69.6875f;
Console.WriteLine(a);
double b = (double)a;
Console.WriteLine("b : {0}",b);
Console.WriteLine("69.6875 == b : {0}", 69.6875 == b);
float x = 0.1f;
Console.WriteLine("x : {0}", x);
double y = (double)x;
Console.WriteLine("y : {0}", y);
Console.WriteLine("0.1 == y : {0}", 0.1 == y);
*/
/*
int a = 500;
Console.WriteLine(a);
uint b = (uint)a;
Console.WriteLine(b);
int x = -30;
Console.WriteLine(x);
uint y = (uint)x;
Console.WriteLine(y);
*/
/*
float a = 0.9f;
int b = (int)a;
Console.WriteLine(b);
float c = 1.1f;
int d = (int)c;
Console.WriteLine(d);
*/
/*
int a = 123;
string b = a.ToString();
Console.WriteLine(b);
float c = 3.14f;
string d = c.ToString();
Console.WriteLine(d);
string e = "123456";
int f = Convert.ToInt32(e);
Console.WriteLine(f);
string g = "1.2345";
float h = float.Parse(g);
Console.WriteLine(h);
*/
/*
const int MAX_INT = 214783647;
const int MIN_INT = -2147483648;
Console.WriteLine(MAX_INT);
Console.WriteLine(MIN_INT);
*/
/*
Console.WriteLine((int)DialogResult.YES);
Console.WriteLine((int)DialogResult.NO);
Console.WriteLine((int)DialogResult.CANCEL);
Console.WriteLine((int)DialogResult.CONFIRM);
Console.WriteLine((int)DialogResult.OK);
*/
/*
DialogResult result = DialogResult.YES;
Console.WriteLine(result == DialogResult.YES);
Console.WriteLine(result == DialogResult.NO);
Console.WriteLine(result == DialogResult.CANCEL);
Console.WriteLine(result == DialogResult.CONFIRM);
Console.WriteLine(result == DialogResult.OK);
*/
/*
Console.WriteLine((int)DialogResult.YES);
Console.WriteLine((int)DialogResult.NO);
Console.WriteLine((int)DialogResult.CANCEL);
Console.WriteLine((int)DialogResult.CONFORM);
Console.WriteLine((int)DialogResult.OK);
*/
/*
int? a = null;
Console.WriteLine(a.HasValue);
Console.WriteLine(a != null);
a = 3;
Console.WriteLine(a.HasValue);
Console.WriteLine(a != null);
Console.WriteLine(a.Value);
*/
/*
var a = 20;
Console.WriteLine("Type : {0}, Value : {1}",a.GetType(),a);
var b = 3.1414213;
Console.WriteLine("Type : {0}, Value : {1}", b.GetType(), b);
var c = "Hello, World";
Console.WriteLine("Type : {0}, Value : {1}", c.GetType(), c);
var d = new int[] {10,20,30};
Console.WriteLine("Type : {0}, Value : ", d.GetType());
foreach (var e in d)
Console.WriteLine("{0} ",e);
Console.WriteLine();
*/
/*
System.Int32 a = 123;
int b = 456;
Console.WriteLine("a type:{0}, value:{1}", a.GetType().ToString(), a);
Console.WriteLine("b type:{0}, value:{1}", b.GetType().ToString(), b);
System.String c = "abc";
string d = "def";
Console.WriteLine("c type:{0}, value:{1}", c.GetType().ToString(), c);
Console.WriteLine("d type:{0}, value:{1}", d.GetType().ToString(), d);
*/
/*
string greeting = "Good Morning";
Console.WriteLine(greeting);
Console.WriteLine();
//indexOf : 지정된 문자 위치 찾기
Console.WriteLine("IndexOf 'Good' : {0} ", greeting.IndexOf("Good"));
Console.WriteLine("IndexOf 'o' : {0} ", greeting.IndexOf("o"));
//LastIndexOf() : 지정된 문자를 뒤에서부터 찾기
Console.WriteLine("LastIndexOf 'Good' : {0}",greeting.LastIndexOf("Good"));
Console.WriteLine("LastIndexOf 'o' : {0}", greeting.LastIndexOf("o"));
//StartsWith() : 문자열로 시작하는지 체크
Console.WriteLine("StartWith 'Good' : {0} :", greeting.StartsWith("Good"));
Console.WriteLine("StartWith 'Morning' : {0} :", greeting.StartsWith("Morning"));
//EndsWith() : 문자열로 끝나는지 체크
Console.WriteLine("EndsWith 'Good' : {0} :", greeting.EndsWith("Good"));
Console.WriteLine("EndsWith 'Morning' : {0} :", greeting.EndsWith("Morning"));
//Contains() : 문자열이 포함되어있는지 체크
Console.WriteLine("Contains 'Evening' : {0} :", greeting.EndsWith("Evening"));
Console.WriteLine("Contains 'Morning' : {0} :", greeting.EndsWith("Morning"));
//Replace() : 치화
Console.WriteLine("Replaced 'Morning' with 'Evening' : {0} :", greeting.Replace("Morning", "Evening"));
*/
/*
Console.WriteLine("ToLower() : '{0}'","ABC".ToLower());
Console.WriteLine("ToLower() : '{0}'", "abc".ToUpper());
Console.WriteLine("Insert() : '{0}'", "Happy Friday!".Insert(5," Sunny"));
Console.WriteLine("Remove() : '{0}'", "I Don't Love You".Remove(2,6));
Console.WriteLine("Trim() : '{0}'", " No Spaces ".Trim() );
Console.WriteLine("TrimStart() : '{0}'", " No Spaces ".TrimStart());
Console.WriteLine("TrimEnd() : '{0}'", " No Spaces ".TrimEnd());
*/
/*
string greeting = "Good Morning";
Console.WriteLine(greeting.Substring(0,5)); //Good
Console.WriteLine(greeting.Substring(5)); //morning
Console.WriteLine();
string[] arr = greeting.Split(new string[] { " " } , StringSplitOptions.None);
Console.WriteLine("Word Count : {0}", arr.Length);
foreach (string element in arr) {
Console.WriteLine("{0}", element);
}
*/
/*
string result = string.Format("'{0}'DEF", "ABC");
Console.WriteLine(result);
string sesult1 = string.Format("'{0,-10}'DEF", "ABC");
Console.WriteLine(sesult1);
string fmt = "'{0,-20}''{1,-15}''{2,30}'";
Console.WriteLine(fmt,"Publisher", "Author", "Title");
Console.WriteLine(fmt, "Marverl", "Stan Lee", "Iron Man");
Console.WriteLine(fmt, "Hanbit", "Sanghyun Park", "This is c#");
Console.WriteLine(fmt, "Prentice Hall", "K&R", "The C Programming Language");
*/
/*
// D : 10 진수
Console.WriteLine("10진수: {0:D}", 123);
Console.WriteLine("10진수: {0:D5}", 123);
// X : 16 진수
Console.WriteLine("16진수: 0x{0:X}", 0xFF1234);
Console.WriteLine("16진수: 0x{0:X8}", 0xFF1234);
// N : 숫자
Console.WriteLine("숫자: {0:N}", 123456789);
Console.WriteLine("숫자: {0:N0}", 123456789);
// F : 고정 소수점
Console.WriteLine("고정 소수점: {0:F}", 123.45);
Console.WriteLine("고정 소수점: {0:F5}", 123.456);
// E : 공학용
Console.WriteLine("공학: {0:E}", 123.456789);
*/
/*
DateTime dt = new DateTime(2023, 06, 28, 23, 18, 22);
Console.WriteLine("12시간 형식: {0:yyyy-MM-dd tt hh:mm:ss (ddd)}", dt);
Console.WriteLine("24시간 형식: {0:yyyy-MM-dd HH:mm:ss (dddd)}", dt);
string aa = string.Format("{0:yyyy-MM-dd HH:mm:ss (dddd)}", dt);
Console.WriteLine(aa);
CultureInfo ciKo = new CultureInfo("ko-KR");
Console.WriteLine();
Console.WriteLine(dt.ToString("yyy-MM-dd tt hh:mm:ss (ddd)", ciKo));
Console.WriteLine(dt.ToString("yyy-MM-dd hh:mm:ss (dddd)", ciKo));
Console.WriteLine(dt.ToString(ciKo));
CultureInfo ciEn = new CultureInfo("en-US");
Console.WriteLine();
Console.WriteLine(dt.ToString("yyy-MM-dd tt hh:mm:ss (ddd)", ciEn));
Console.WriteLine(dt.ToString("yyy-MM-dd hh:mm:ss (dddd)", ciEn));
Console.WriteLine(dt.ToString(ciEn));
*/
string name = "김튼튼";
int age = 23;
Console.WriteLine($"{name,-10},{age:D3},{"'aa'":5}");
name = "박날씬";
age = 30;
Console.WriteLine($"{name}, {age,-10:D3}");
name = "이비실";
age = 17;
Console.WriteLine($"{name}, {(age > 20 ? "성인" : "미성년자")}");
}
}
}
'CSharp > CSharp 문법' 카테고리의 다른 글
메소드 ( Method) (0) | 2024.08.16 |
---|---|
코드의 흐림제어 (0) | 2024.08.01 |
C# 강의 메모 (0) | 2022.09.28 |
기초문법 정리2 (0) | 2022.09.27 |
두 문자열 경로로 결합 (0) | 2022.09.26 |
댓글